Delphix RESTFul APIs command line basics
Authentication
RESTFul APIs require authentication. Just plugging the URL into a web browser or running an operating system cURL command will return an authentication/login required error message.
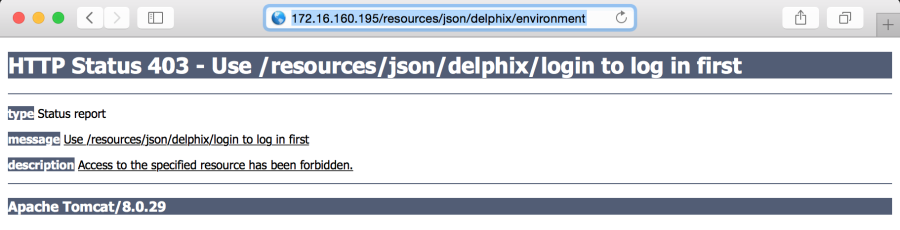
Command line:
curl http://172.16.160.195/resources/json/delphix/environment
Response:
<!DOCTYPE html><html><head><title>Apache Tomcat/8.0.29 - Error report</title><style type="text/css">H1 {font-family:Tahoma,Arial,sans-serif;color:white;background-color:#525D76;font-size:22px;} H2 {font-family:Tahoma,Arial,sans-serif;color:white;background-color:#525D76;font-size:16px;} H3 {font-family:Tahoma,Arial,sans-serif;color:white;background-color:#525D76;font-size:14px;} BODY {font-family:Tahoma,Arial,sans-serif;color:black;background-color:white;} B {font-family:Tahoma,Arial,sans-serif;color:white;background-color:#525D76;} P {font-family:Tahoma,Arial,sans-serif;background:white;color:black;font-size:12px;}A {color : black;}A.name {color : black;}.line {height: 1px; background-color: #525D76; border: none;}</style> </head><body><h1>HTTP Status 403 - Use /resources/json/delphix/login to log in first</h1><div class="line"></div><p><b>type</b> Status report</p><p><b>message</b> <u>Use /resources/json/delphix/login to log in first</u></p><p><b>description</b> <u>Access to the specified resource has been forbidden.</u></p><hr class="line"><h3>Apache Tomcat/8.0.29</h3></body></html>
The authentication process requires you to establish a session first.
The example within this section illustrates the session/login and subsequent API calls with cURL using cookies created when the session was established.
Session
When programming for compatibility, the API version number is very important. Please be aware of the differences between versions for enterprise applications. For example, if you specify a 1.11.6 version, ONLY the available calls and functionality for that version will be used, and it will only be operational on Delphix Engine versions that support that version.
The session uses the –c for the cookie creation, the login uses the -b for the exiting cookie created by the session, and -c to create a new cookie, the other commands use the –b for using the existing cookie created by the login.
From the Unix/Linux command line
$ curl -s -X POST -k --data @- http://delphix-server/resources/json/delphix/session \ -c ~/cookies.txt -H "Content-Type: application/json" <<EOF{ "type": "APISession", "version": { "type": "APIVersion", "major": 1, "minor": 11, "micro": 6 }}EOF
Returned to the command line are the results (added linefeeds for readability)
{ "status":"OK", "result": { "type":"APISession", "version": { "type": "APIVersion", "major": 1, "minor": 11, "micro": 6 }, "locale": "en_US", "client": null }, "job": null}
Login
Once you have established the session, the next step is to authenticate to the server by executing the Login Request API. Unauthenticated sessions are prohibited from making any API calls other than this login request. The username can be either a system user or domain user; the backend will authenticate using the appropriate method.
The session uses the –c for the cookie creation, the login uses the -b for the exiting cookie created by the session, and -c to create a new cookie, the other commands use the –b for using the existing cookie created by the login.
From the Unix/Linux command line
$ curl -s -X POST -k --data @- http://delphix-server/resources/json/delphix/login \ -b ~/cookies.txt -c ~/cookies.txt -H "Content-Type: application/json" <<EOF{ "type": "LoginRequest", "username": "delphix_username", "password": "delphix_password"}EOF
Returned to the command line are the results (added linefeeds for readability)
{"status":"OK","result":"USER-2","job":null,"action":null}
Sample Delphix API call
With a successful authentication (session, login, and saved cookie), calls to the Delphix Engine can now be made to perform the desired functionality.
The session uses the –c for the cookie creation, the login uses the -b for the exiting cookie created by the session, and -c to create a new cookie, the other commands use the –b for using the existing cookie created by the login.
For starters, let's create a session, login, and get the existing environments defined within the Delphix Engine.
curl -s -X POST -k --data @- http://172.16.160.195/resources/json/delphix/session \-c ~/cookies.txt -H "Content-Type: application/json" <<EOF{ "type": "APISession", "version": { "type": "APIVersion", "major": 1, "minor": 7, "micro": 0 }}EOF
curl -s -X POST -k --data @- http://172.16.160.195/resources/json/delphix/login \ -b ~/cookies.txt -c ~/cookies.txt -H "Content-Type: application/json" <<EOF{ "type": "LoginRequest", "username": "admin", "password": "delphix"}EOF
curl -X GET -k http://172.16.160.195/resources/json/delphix/environment \ -b ~/cookies.txt -H "Content-Type: application/json"
Returned to the command line are the results (added linefeeds for readability)
{"type":"ListResult","status":"OK","result":[ {"type":"WindowsHostEnvironment", "reference":"WINDOWS_HOST_ENVIRONMENT1", "namespace":null, "name":"Window Target", "description":"", "primaryUser":"HOST_USER-1", "enabled":false, "host":"WINDOWS_HOST1", "proxy":null }, { "type":"UnixHostEnvironment", "reference":"UNIX_HOST_ENVIRONMENT-3", "namespace":null, "name":"Oracle Target", "description":"", "primaryUser":"HOST_USER-3", "enabled":true, "host":"UNIX_HOST-3","aseHostEnvironmentParameters":null }],"job":null,"action":null,"total":2,"overflow":false}
Windows PowerShell authentication example
See the PowerShell section below if cURL is not yet available on your operating system.
These commands work on Windows Command Prompt with the respective JSON files: session.json and login.json
Filename: session.json
{ "type": "APISession", "version": { "type": "APIVersion", "major": 1, "minor": 7, "micro": 0 }}
Filename: login.json
{"type": "LoginRequest","username": "admin","password": "delphix"}
(In Powershell you use curl.exe or modify the default alias)...
curl.exe --insecure -c cookies.txt -sX POST -H "Content-Type: application/json" -d "@session.json" http://172.16.160.195/resources/json/delphix/sessioncurl.exe --insecure -b cookies.txt -c cookies.txt -sX POST -H "Content-Type: application/json" -d "@login.json" http://172.16.160.195/resources/json/delphix/logincurl.exe --insecure -b cookies.txt -sX GET -H "Content-Type: application/json" -k http://172.16.160.195/resources/json/delphix/system
Putting the above commands within a Powershell script:
Filename: auth1.ps1
<#Filename: auth.ps1Description: Delphix Powershell Sample Authentication Script ...Date: 2016-08-02Author: Bitt...#>
Variables ...
$nl = [Environment]::NewLine$BaseURL = "http://172.16.160.195/resources/json/delphix"$cookie = "cookies.txt"
Session JSON Data ...
write-output "${nl}Creating session.json file ..."$json = @"{ "type": "APISession", "version": { "type": "APIVersion", "major": 1, "minor": 11, "micro": 6 }}"@
Output File using UTF8 encoding ...
write-output $json | Out-File "session.json" -encoding utf8
Delphix cURL Session API ...
write-output "${nl}Calling Session API ...${nl}"$results = (curl.exe --insecure -c "$cookie" -sX POST -H "Content-Type: application/json" -d "@session.json" -k $BaseURL/session)write-output "Session API Results: ${results}"
Login JSON Data ...
write-output "${nl}Creating login.json file ..."$user = "admin"$pass = "delphix"$json = @"{ "type": "LoginRequest", "username": "${user}", "password": "${pass}"}"@
Output File using UTF8 encoding ...
write-output $json | Out-File "login.json" -encoding utf8
Delphix cURL Login API ...
write-output "${nl}Calling Login API ...${nl}"$results = (curl.exe --insecure -b "$cookie" -c "$cookie" -sX POST -H "Content-Type: application/json" -d "@login.json" -k $BaseURL/login)write-output "Login API Results: ${results}"
Delphix cURL system API ...
write-output "${nl}Calling System API ...${nl}"$results = (curl.exe --insecure -b "$cookie" -sX GET -H "Content-Type: application/json" -k $BaseURL/system)write-output "System API Results: ${results}"
The end is near ...
echo "${nl}Done ...${nl}"exit;
Sample Powershell Script Output:
PS> . .\auth.ps1Creating session.json file ...Calling Session API ...Session API Results:{"type":"OKResult","status":"OK","result":{"type":"APISession","version":{"type":"APIVersion","major":1,"minor":7,"micro":0},"locale":null,"client":null},"job":null,"action":null}Creating login.json file ...Calling Login API ...Login API Results: {"type":"OKResult","status":"OK","result":"USER2","job":null,"action":null}Calling System API ... System API Results: {"type":"OKResult","status":"OK","result":{"type":"SystemInfo","productType":"standard","productName":"Delphix Engine","buildTitle":"Delphix Engine 5.1.1.0","buildTimestamp":"20160721T07:23:41.000Z","buildVersion":{"type":"VersionInfo","major":5,"minor":1,"micro":1,"patch":0},"configured":true,"enabedFeatures":["XPP","MSSQLHOOKS"],"apiVersion":{"type":"APIVersion","major":1,"minor":8,"micro":0},"banner":null,"locals":["enUS"],"currentLocale":"enUS","hostname":"Delphix5110HWv8","sshPublicKey":"ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQDOsrp7Aj6hFQh9yBq7273B+qtPKmCu1B18nPvr08yjt/IZeM4qKk7caxExQS9rpfU8AWoT7e8ESV7NkBmUzOHrHnLsuJtxPqeYoqeMubVxYjJuxlH368sZuYsnB04KM0mi39e15lxVGvxQk9tyMpl7gs7cXRz1k6puncyiczU/axGq7ALHU2uyQoVmlPasuHJbq23d21VAYLuscbtgpZLAFlR8eQH5Xqaa0RT+aQJ6B1ihZ7S0ZN914M2gZHHNYcSGDWZHwUnBGttnxx1ofRcyN4/qwT5iHq5kjApjSaNgSAU0ExqDHiqgTq0wttf5nltCqGMTFR7XY38HiNq++atDroot@Delphix5110HWv8\n","memorySize":8.58107904E9,"platform":"VMware with BIOS date 05/20/2014","uuid":"564d7e1df4cb-f91098fd348d74817683","processors":[{"type":"CPUInfo","speed":2.5E9,"cores":1}],"storageUsed":2.158171648E9,"storageTotal":2.0673724416E10,"installationTime":"2016-07-27T13:28:46.000Z"},"job":null,"action":null} Done ... PS>